Bubble Sort – Python
Introduction
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted. Here’s a simple tutorial on implementing Bubble Sort in Python.
Bubble sort is also known as sinking sort or comparison sort. In bubble sort each element is compared with the adjacent element, and the elements are swapped if they are found in wrong order. However this is a time consuming algorithm. It is simple but quite inefficient.
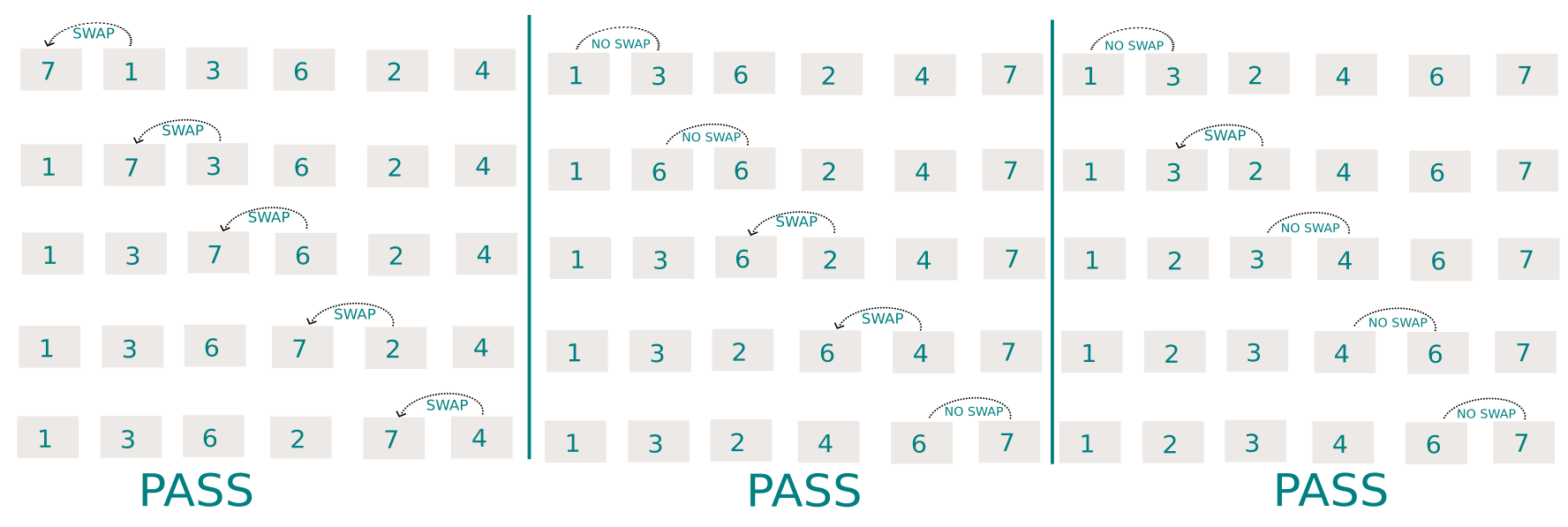
Implementation of Bubble Sort
The code for a double sort algorithm is very.
Step 1
Define the function for bubble sort. It would take the list that needs to be sorted as input.
def bubble_sort(input_list):
Step 2
- Set a loop
for i in range len(input_list)
- Inside this for loop set another loop
for j in range len(input_list)-i-1)
. - For every
i
in the nested loop value at indexj
is compared with value at indexj+1
. If the value at indexj+1
is smaller than the value at indexj
then the values are swapped. - After the for loop is over print the sorted list.
def bubble_sort(input_list):
for i in range(len(input_list)):
for j in range(0, len(input_list) - i - 1):
if input_list[j] > input_list[j + 1]:
temp = input_list[j]
input_list[j] = input_list[j+1]
input_list[j+1] = temp
Execution
data = [2, 45, 0, 11, -9]
print("Sorted Input List in ascending Order:", data)
bubble_sort(data)
print(data)
data_two = [45, 80, 4, 5, 1, 0]
print("Executing Bubble sort for", data_two)
bubble_sort(data_two)
print(data_two)
Output
Sorted Input List in ascending Order: [2, 45, 0, 11, -9]
[-9, 0, 2, 11, 45]
Executing Bubble sort for [45, 80, 4, 5, 1, 0]
[0, 1, 4, 5, 45, 80]