In computer science data types are very important and basic concept that represents the nature of data that can be handle within a computer program.
Python data types are the classification and grouping of data items. It describe the type of value that tells what operations can be performed on a particular data.
Python has default Built-in data types in these category
Category | Python Data Types |
---|---|
Text Type: | str |
Numeric Types: | int , float , complex |
Sequence Types: | list , tuple , range |
Mapping Type: | dict |
Set Types: | set , frozenset |
Boolean Type: | bool |
Binary Types: | bytes , bytearray , memoryview |
None Type: | NoneType |
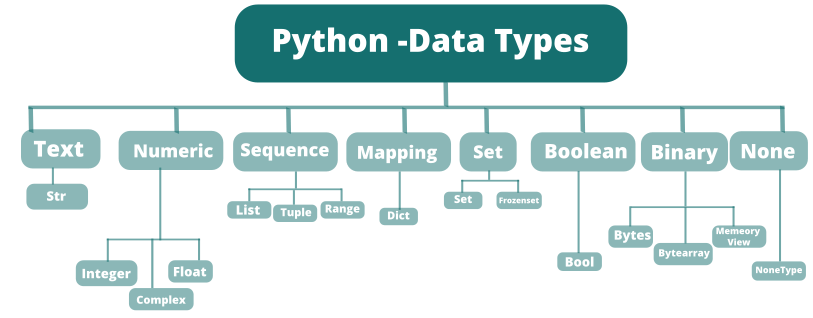
How to get data type in Python
We can use Python Built-in function type()
to get the dat type of any objects.
a = "codewithronny.com"
print(type(a))
Why do we set data types
In Python data types automatically set when user assign a value to variables. There is no need to declare type of variable in Python.
Sample Code | Data Types | Run |
---|---|---|
a = "Hello Freaks" | str | Run |
a = 40 | int | Run |
a = 540.5 | float | Run |
a = 1z | complex | Run |
a = ["Protein", "Calcium", "Minerals"] | list | Run |
a = (" | tuple | Run |
a = {"name" : "Ronny", "age" : 27} | dictionary | Run |
a = {" | set | Run |
a = frozenset({" | frozenset | Run |
a = range(10) | range | Run |
a = True | boolean | Run |
a = b"Hello" | bytes | Run |
a = bytearray(8) | bytearray | Run |
a = memoryview(bytes(20)) | memoryview | Run |
a = None | None Type | Run |