How to create professional lead collecting form for website
Last updated: September 13, 2024 By Sunil Shaw
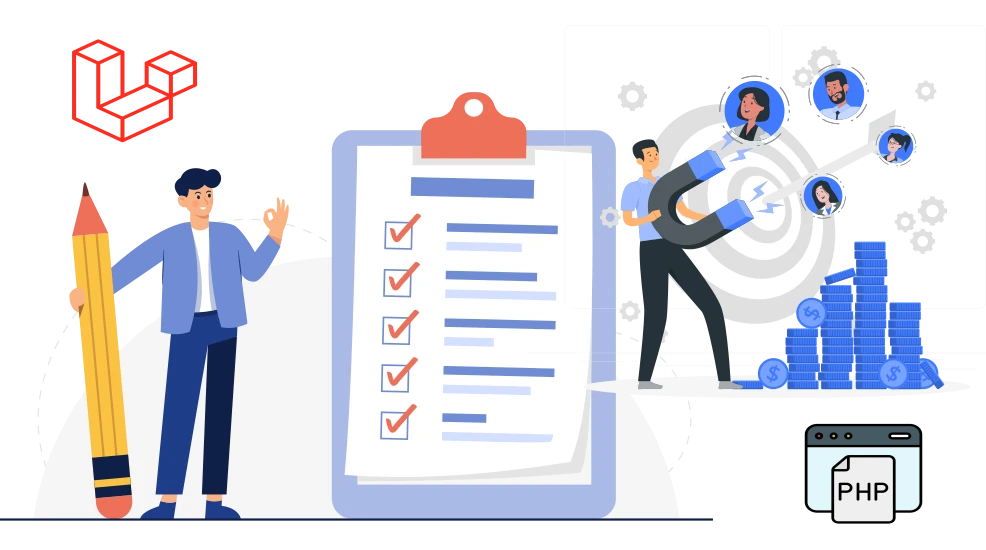
Any company needs leads to increase sells and company growth. So it’s important to have the lead collecting form on your client website. In this article we will learn How to create professional lead collecting form for website. We will see how to develop an web page using PHP Laravel and Mysql to store all the leads in database. Previously i were developing this project for one of my client Luisnvaya. I created this form to collect customer enquiry as a lead and display in Admin area.
What is Laravel framework and why is it used?
Laravel is an easy-to-use open-source PHP framework for developing web projects like websites and applications. It gives features and rich library to develop backend and frontend of any web applications. There is crucial part to take decisions before going to start any project, to choose which language and library would be easy and future friendly. Being a developer i suggets to choose Laravel framework. Laravel is an cross-platform open-source php framework with huge extensive library and pre-programmed functionalities such as HTML templating, routing and authentication. Laravel is an powerful framework on Model-View-Controller(MVC) architecture.
Is Laravel backend or frontend?
Specially Laravel is a backend framework that provides all of the features you need to build modern web applications, such as routing, validation, caching, queues, file storage, and more, But you can also build your frontend into it. Sepcially being a developer is used most of the time Laravel as a full stack development for our web projects.
How to create professional lead collecting form for website
Lets see how to create an form.
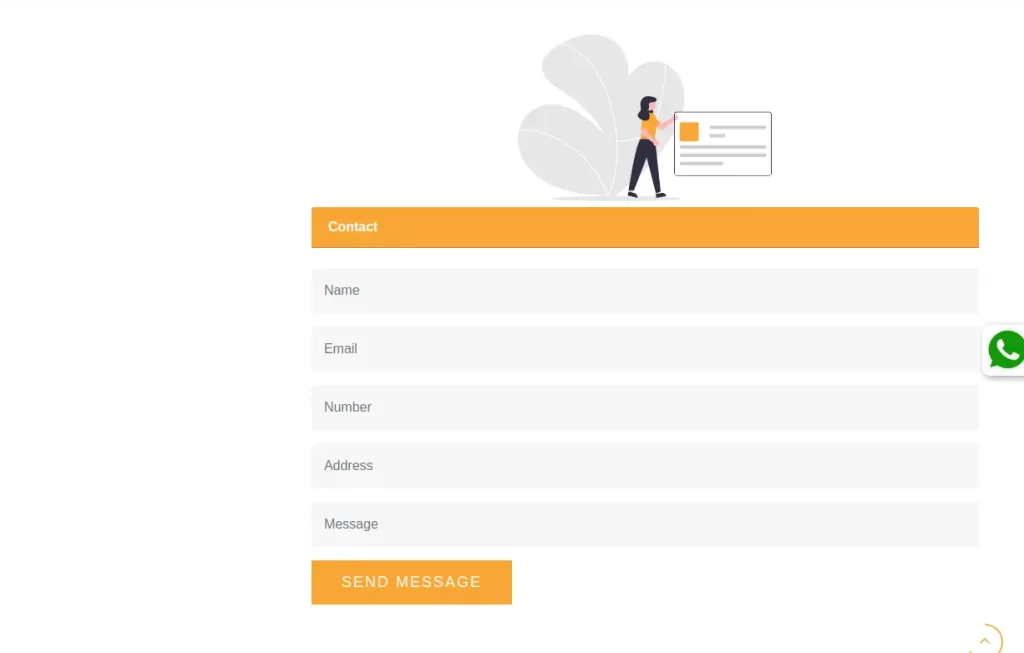
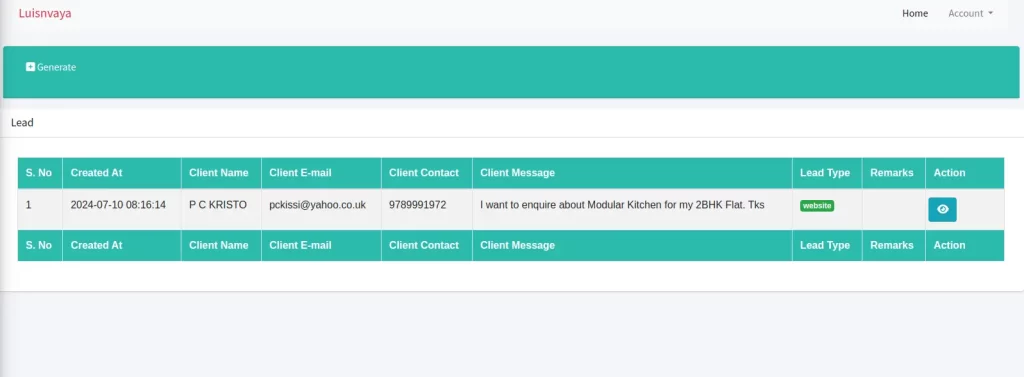
Make Routes
Route::get('/contact-us', [App\Http\Controllers\ContactController::class, 'contactUs'])->name('contact_us');
Route::post('/lead-save', [App\Http\Controllers\Frontend\HomeController::class, 'LeadSave'])->name('lead_save');
Let’s make two routes one for leads collecting page and another for storing the leads. The first route contact-us
for loading contact form and second route lead-save
for storing the lead in database.
Create a Controller
So next step is to create a contoller named ContactController
in app/Http/Controllers/ContactController
. In Laravel controller is an PHP class that act as controlling system for an web application request-response cycle. It contains functions that handle lots of HTTP request. Developer can define custom number of function within a controller.
Here is an example your controller code should look like this.
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
class ContactController extends Controller
{
}
Now create a function named ContactUs
to serve the html page.
public function ContactUs()
{
return view('contact_us');
}
Create an another function EnquireSave
to save the enquiries or leads into database.
public function EnquirySave(Request $request)
{
$data = $request->all();
$lead = Lead::create([
'name' => $data['name'],
'email' => $data['email'],
'contact' => $data['contact'],
'address' => $data['address'],
'message' => $data['message'],
'lead_type' => "website",
'client_status' => "off",
'viewed' => 0,
'remarks' => "",
'status' => 1
]);
if(isset($lead)) {
return redirect()->back()->with('success','Thank you we are on the way to contact you.');
}else{
return redirect()->back()->with('success','Error: Your action Failed Please try again.');
}
}
Full Code is
<?php
namespace App\Http\Controllers;
use App\Models\Lead;
use Illuminate\Http\Request;
use App\Http\Requests\PostRequest;
use Illuminate\Support\Facades\Cache;
use App\Http\Controllers\Controller;
class ContactController extends Controller
{
public function ContactUs()
{
return view('contact_us');
}
public function EnquirySave(Request $request)
{
$data = $request->all();
$lead = Lead::create([
'name' => $data['name'],
'email' => $data['email'],
'contact' => $data['contact'],
'address' => $data['address'],
'message' => $data['message'],
'lead_type' => "website",
'client_status' => "off",
'viewed' => 0,
'remarks' => "",
'status' => 1
]);
if(isset($lead)) {
return redirect()->back()->with('success','Thank you we are on the way to contact you.');
}else{
return redirect()->back()->with('success','Error: Your action Failed Please try again.');
}
}
}
Creates Database

CREATE TABLE `lead` (
`id` bigint UNSIGNED NOT NULL,
`name` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`email` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`contact` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`address` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`message` text CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`lead_type` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`client_status` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`viewed` int NOT NULL DEFAULT '0',
`remarks` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`status` int NOT NULL,
`created_at` timestamp NULL DEFAULT NULL,
`updated_at` timestamp NULL DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
ALTER TABLE `lead`
ADD PRIMARY KEY (`id`);
ALTER TABLE `lead`
MODIFY `id` bigint UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=3;
COMMIT;
Insert data into lead table.
INSERT INTO `lead` (`id`, `name`, `email`, `contact`, `address`, `message`, `lead_type`, `client_status`, `viewed`, `remarks`, `status`, `created_at`, `updated_at`) VALUES
(2, 'Ronny Dsouzaffffff', 'codewithronny@gmail.com', '1234567890', '5/22 lig colony vidya nagar Bhopalf', 'sdxfffffff', 'site scanfffffff', '0f', 1, 'gfdf', 1, '2023-11-05 05:47:27', '2023-11-18 00:52:08');
Full Script to create database and insert data into it.
CREATE TABLE `lead` (
`id` bigint UNSIGNED NOT NULL,
`name` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`email` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`contact` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`address` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`message` text CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`lead_type` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`client_status` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`viewed` int NOT NULL DEFAULT '0',
`remarks` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`status` int NOT NULL,
`created_at` timestamp NULL DEFAULT NULL,
`updated_at` timestamp NULL DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
--
-- Dumping data for table `lead`
--
INSERT INTO `lead` (`id`, `name`, `email`, `contact`, `address`, `message`, `lead_type`, `client_status`, `viewed`, `remarks`, `status`, `created_at`, `updated_at`) VALUES
(2, 'Ronny Dsouzaffffff', 'codewithronny@gmail.com', '1234567890', '5/22 lig colony vidya nagar Bhopalf', 'sdxfffffff', 'site scanfffffff', '0f', 1, 'gfdf', 1, '2023-11-05 05:47:27', '2023-11-18 00:52:08');
--
-- Indexes for dumped tables
--
--
-- Indexes for table `lead`
--
ALTER TABLE `lead`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `lead`
--
ALTER TABLE `lead`
MODIFY `id` bigint UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=3;
COMMIT;
Creates Model
Lets connect with database using Lead
model.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Lead extends Model
{
use HasFactory;
protected $table = "lead";
protected $fillable = [ 'id', 'name', 'email', 'contact','address','message','lead_type','client_status','viewed','remarks','status'];
}
Create View
Let’s create html template and input to store data. You can see this below form, here we using bootstrap CDN for bootstrap support and combination with html.
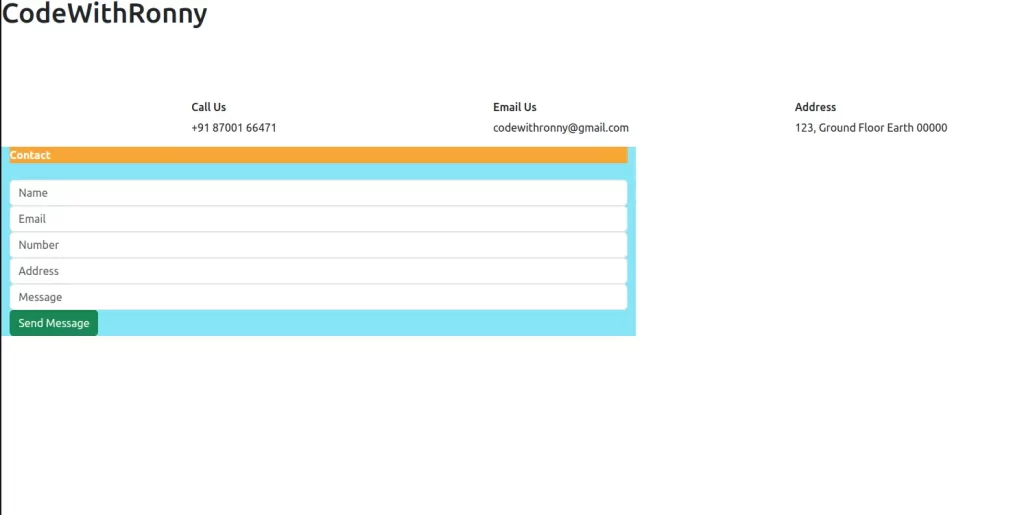
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>HTML Bootstrap CDN Based Biolerplate</title>
<meta name="viewport" content="width=device-width,initial-scale=1" />
<meta name="description" content="" />
<link rel="icon" href="favicon.png">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css">
</head>
<body>
<h1>CodeWithRonny</h1>
<!-- ==================== Start about ==================== -->
<br>
<br>
<br>
<br>
<section class="contact">
<div class="info bg-gray pt-80 pb-80">
<div class="container">
<div class="row">
<div class="col-lg-4">
<div class="item">
<span class="icon pe-7s-phone"></span>
<div class="cont">
<h6 class="custom-font">Call Us</h6>
<p>+91 87001 66471</p>
</div>
</div>
</div>
<div class="col-lg-4">
<div class="item">
<span class="icon pe-7s-mail-open"></span>
<div class="cont">
<h6 class="custom-font">Email Us</h6>
<p>codewithronny@gmail.com</p>
</div>
</div>
</div>
<div class="col-lg-4">
<div class="item">
<span class="icon pe-7s-map"></span>
<div class="cont">
<h6 class="custom-font">Address</h6>
<p> 123, Ground Floor Earth 00000</p>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="container-fluid">
<div class="row">
<div class="col-md-6 bg-info bg-opacity-50">
<div class="form ">
<form id="" method='POST' enctype="multipart/form-data" action="{{ route('lead_save') }}">
@csrf
<div class="messages"></div>
<div class="controls">
<div class="card-header" style="background-color:#f9a735; color:#ffff">
<b>Contact</b>
</div></br>
<div class="form-group">
<input type="text" name="name" placeholder="Name" class="form-control" required>
<!-- <input id="form_name" type="text" name="name" placeholder="Name" required="required"> -->
</div>
<div class="form-group">
<input type="email" name="email" placeholder="Email" class="form-control" required>
</div>
<div class="form-group">
<input type="number" name="contact" placeholder="Number" class="form-control" required>
</div>
<div class="form-group">
<input type="text" name="address" placeholder="Address" class="form-control" required>
</div>
<div class="form-group">
<input type="text" name="message" placeholder="Message" class="form-control" required>
</div>
<button type="submit" class="btn btn-success"><span>Send Message</span></button>
</div>
</form>
</div>
</div>
</div>
</div>
</section>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.min.js"></script>
</body>
</html>
How to Create an Professional Blog Using Laravel PHP
Conclusions
So i hope you learn How to create professional lead collecting form for website. If you want to create an professional blog Read this article.
About Author
I am a Web Developer, Love to write code and explain in brief. I Worked on several projects and completed in no time.
View all posts by Sunil Shaw