Creating Python Function That Take Parameters
Last updated: September 13, 2024 By Sunil Shaw
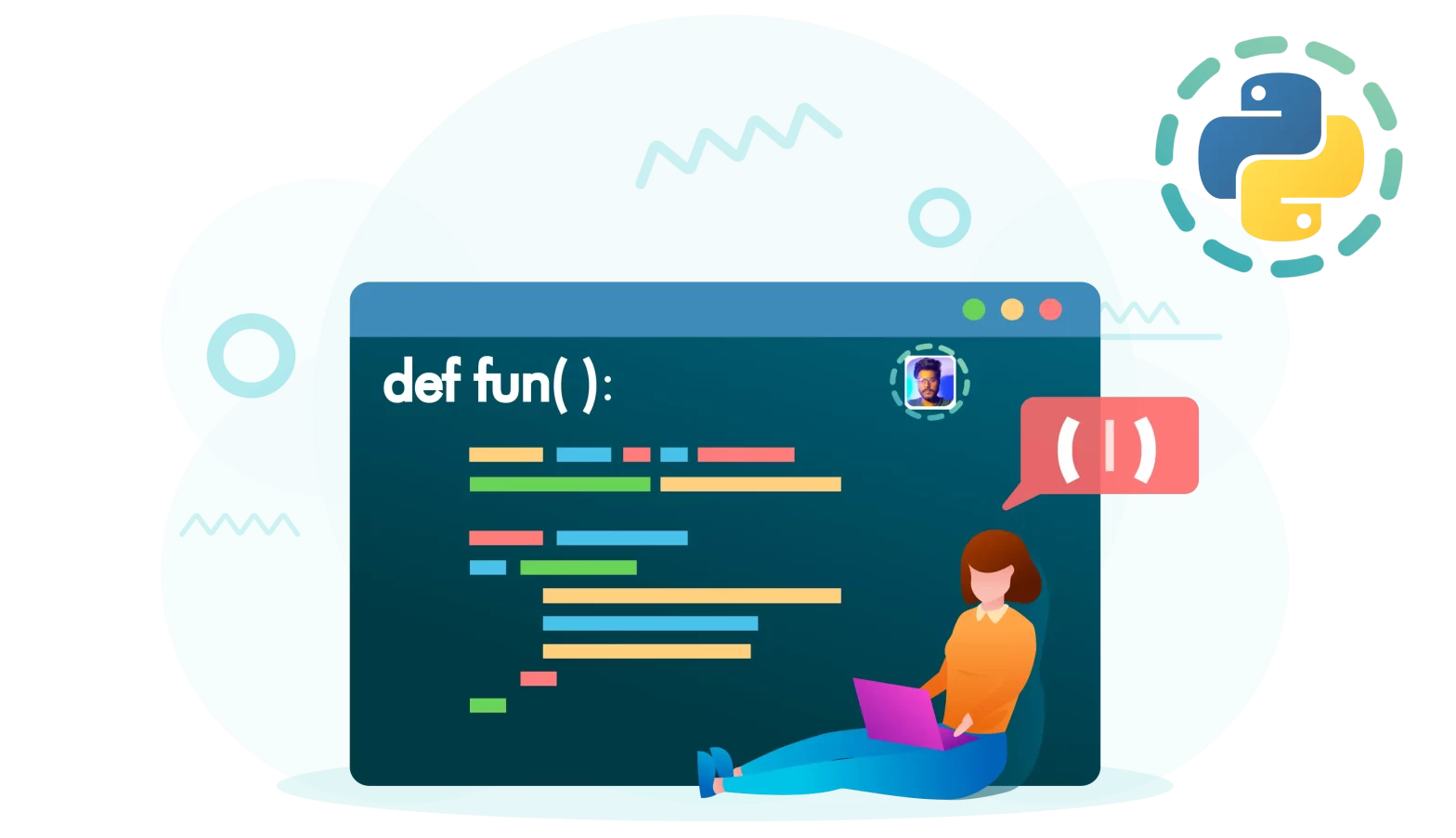
Introduction
According to Wikipedia Python is a high-level and general-purpose programming language. Its focus on design code readability with the use of significant indentation. Python dynamically typed language and garbage collected. It supports multiple programming mthod, including structured, object-oriented and functional programming. Read more about python on Wikipedia.Function in python is an block of code or blueprint of code. In this tutorial we will learn how to creating python function that take parameters.
Programmers organize code into reusable blocks called functions. In Python functions are important.
- Function provides better readability and modularity.
- Functions help us to save time and hard work in designing and executing the code.
- It reduces to getting chance of duplication of code.
- It makes code resuable.
- Function make code easy to maintainable.
In Python programming language, functions are classified mainly in two category.
- Built-in Functions: It is an function that are provided by Python by default.
- User-defined Functions: In this articles we will learn how to create function and pass parameters into it. Programmers creates this function to perform a tasks.
Let’s See Creating Python function that take parameters
You will create your first function. For creating a function, you will have to follow these rules
- How to create a function
- Create parameter and pass arguments into it
- The function body
- Calling function
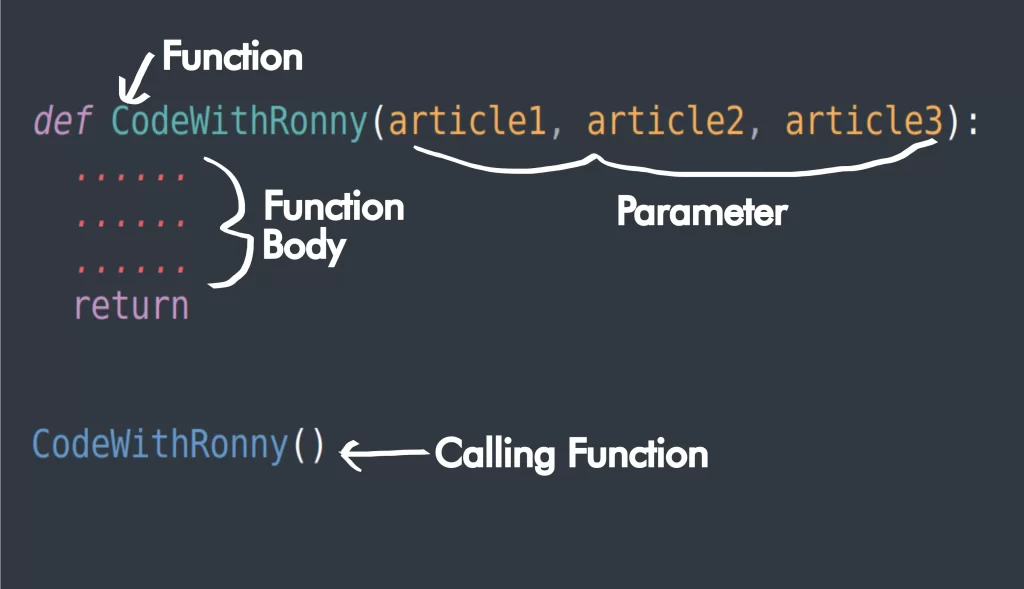
Creation of a function
- The creation of a function starts with the
def
keyword. - After defining
def
keyword, name of function are defined. - After name of the function parenthesis
( )
are defined. - We define parameters within parenthesis.
- After the parenthesis colon
:
comes. Colon indicates the begning of the function’s block of code.
def CodeWithRonny():
Overall, in above code we are creating an function called CodeWithRonny
.
Function Body
To clarify, the function body is an block of code that comes after the function definition. According to PEP8 indentation should be one level to the right, Similarly shown in above figure 2. In other words function body used to define code logic and conditions to be return after execution.
def CodeWithRonny():
# every code logic written there
i_am_a_variable = "You wrote me in function body"
return i_am_a_variable
In summary, we are creating an function, Just as created before, in next line defining variable called i_am_a_variable
and storing String
value. Finally retuning that variable. After calling the function it will return “You wrote me in function body” as a result. However move to next point.
Calling a function
In a word, firstly you can call a function anytime and secondly call the function by its name follow by parenthesis. First thing to remember function does not execute as long as called. For that reason call the function by it’s name to execute. If you call two times undoubtedly it will execute two times. However it will print result two times.
CodeWithRonny()
Overall you learned how to creates a function. Whenever now move to another tutorial to learn pass arguments into a function.
Creating Function That Take Parameters
Previously we learned to create function and call them, then again we will take same function to take parameter.
def CodeWithRonny(website):
return website
We created an function called CodeWithRonny
and putting parameter website
into parenthesis then returns the parameter.
Note: Remember we created an function with parameter, so whenever calling the function, we have to pass the argument into it. Here codewithronny.com
is an argument, you can pass your own.
CodeWithRonny("codewithwonny.com")
Finally calling the function to print the executed message. Here below is full code.
def CodeWithRonny(website):
return website
CodeWithRonny("codewithwonny.com")
Proceed to the next step before becoming frustrated. Undoubtedly code executed successfully but only on console not on screen. You have to use print()
built-in function to print the message in human readable form on screen.
def CodeWithRonny(website):
return website
print(CodeWithRonny("codewithwonny.com"))
Output:
codewithwonny.com
[Finished in 13ms]
Without a doubt you got an output like above, congratulations you wrote your first Python function with parameter successfully.
Create function with three parameter
In short, we will take same function and put three parameters into it. Afterward call the function with three arguments.
def CodeWithRonny(website1, website2, website3):
return website1, website2, website3
print(CodeWithRonny("codewithwonny.com", "editor.codewithronny.com", "forum.codewithronny.com"))
Output:
('codewithwonny.com', 'editor.codewithronny.com', 'forum.codewithronny.com')
[Finished in 17ms]
Note: It will return tuple of website.
Here we can use different approach to print result in different line.
def CodeWithRonny(website1, website2, website3):
print(website1)
print(website2)
print(website3)
CodeWithRonny("codewithwonny.com", "editor.codewithronny.com", "forum.codewithronny.com")
Output:
codewithwonny.com
editor.codewithronny.com
forum.codewithronny.com
[Finished in 15ms]
We are not calling function within print
function, because we used print
function already inside our function. While using return in a function, it returns object, so we can use print function to catch that object in human readable form.
What is Python Functional Arguments
There are five type of python functional arguments.
- Positional Arguments
- Default Arguments
- Keyword Arguments
- *args
- **kwargs
In this article we will cover three type of arguments, positional, default and keyword arguments.
Positional Arguments
Arguments are assigned to the parameters in the order in their position.
def CodeWithRonny(number1, number2):
sum_of_number = number1+number2
product_of_number = number1*number2
return sum_of_number, product_of_number
x = int(input('Enter the first number :'))
y = int(input('Enter the second number :'))
print(CodeWithRonny(x, y))
Output
Enter the first number : 20
Enter the second number : 30
(230, 6000)
[Finished in 15ms]
In function code looks at the position of the parameter where it will be assigned. So, the value of X gets mapped to number1
, and value of the y gets mapped to number2
and these values passed on to the function block code.
If you call a function with different number of parameters, then, it will through an error.
Default Arguments
You have the option of specifying default value of a parameter in the function definition. The positional arguments for which a default value is defined, becomes optional and therefore known as default arguments.
def Customer(first_name, last_name ='Dsouza', standard ='Delhi'):
print(first_name, last_name, 'lives in', standard, 'Standard')
Calling the function
def Customer(first_name, last_name ='Dsouza', standard ='Delhi'):
print(first_name, last_name, 'lives in', standard, 'Standard')
# 1 positional argument
Customer('Ronny')
# 3 positional arguments
Customer('Bhawna', 'Bairagi', 'Raipur')
# 2 positional arguments
Customer('Sagar', 'Rawat')
Customer('Deepika', 'Mandana')
Output
Ronny Dsouza lives in Delhi Standard
Bhawna Bairagi lives in Raipur Standard
Sagar Rawat lives in Delhi Standard
Deepika Mandana lives in Delhi Standard
[Finished in 15ms]
Another example code:
def sum_prod(number1, number2=0):
sum_of_number = number1 + number2
product_of_number = number1 * number2
return sum_of_number, product_of_number
print(sum_prod(3,5))
print(sum_prod(3))
Output
(8, 15)
(3, 0)
[Finished in 13ms]
One or two arguments can call the function shown above. If you omit the second argument, the function definition will passon its default value, which is 0
.
Let’s take a look at another example.
In Python, a non-default aguments cannot follow a default arguments.
def sum_func(number1, number2=0, number3):
return number1+number2+number3
The preceding function will throw an error because number3
, which is an non-default arguments follows number2
, which is a default argument. So, if you type sum_func(10, 20)
, the interpreter will not undderstand whether to assign 20 to number2
or continue with default value. As the number of default arguments increases, the complexity will increase. In this scenario you will receive a Syntax Error. “non default arguments follow default arguments”. The below code shows the correct way of using default arguments.
def sum_func(number1, number2=10, number3=20):
return number1 + number2 + number3
print(sum_func(20))
print(sum_func(20, 40))
print(sum_func(10, 20, 30))
Output
50
80
60
[Finished in 13ms]
Keyword arguments
Keyword arguments enable ignoring the order in which you enter parameters in a function or even skipping them when you call a function
The function with keyword arguments defines in the same way as the function with positional arguments, but the difference lies in the way they call it. Have a look at the following code.
def sum_func(number1, number2=10, number3=20):
print("number1 = ", number1)
print("number2 = ", number2)
print("number3 = ", number3)
return number1 + number2 + number3
print(sum_func(number3=10, number1=20))
Output
number1 = 20
number2 = 10
number3 = 10
40
[Finished in 13ms]
You can observe that the arguments don’t follow the desired order, but while passing them, you specify which arguments belong to which parameter. Since the default value of number2 is zero, skipping it doesn’t matter.We wanted to use the default value of number2 therefore only the value of number2 and number3 were specified. If you don’t do that, the output will be incorrect. In the following code, we assign the value 20 to number2, and the function takes the default value of number3, resulting in a completely different outcome.
number1 = 20
number2 = 10
number3 = 10
40
[Finished in 13ms]
Output
number1 = 10
number2 = 20
number3 = 40
70
About Author
I am a Web Developer, Love to write code and explain in brief. I Worked on several projects and completed in no time.
View all posts by Sunil Shaw