How to Create an Professional Blog Using Laravel PHP
Last updated: February 22, 2025 By Sunil Shaw
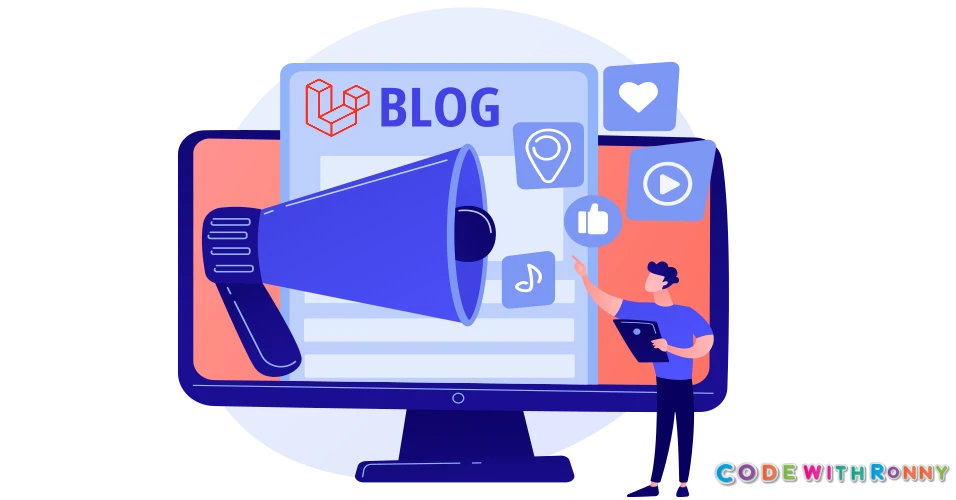
Introduction
Laravel is an PHP’s free open source and famous web framework, developed by Taylor Otwell to develop web application for MVC (Model View Controller) architectural patterns. It supports backend and frontend development. Let’s see How to Create an Professional Blog Using Laravel PHP.
How To Create Dynamic Slider Using Laravel Step By Step
How to Create an Professional Blog Using Laravel PHP
In this article we will learn how to develop blog using laravel. Right now i am creating blogging system for one of my client Luisnvaya. There is more need of blog section on every website, make sure you are creating proferssional blogging system for your own website or for your client website. Here we will see how an professional blog creates.
Let’s build a new laravel app named blog
. Use below command to create new project in current directory.
composer create-project laravel/laravel blog
Let’s run laravel app to check installation completed successfully.
php artisan serve
Check Laravel App In Browser
go to your browser and hit the localhost http://127.0.0.1:8000/
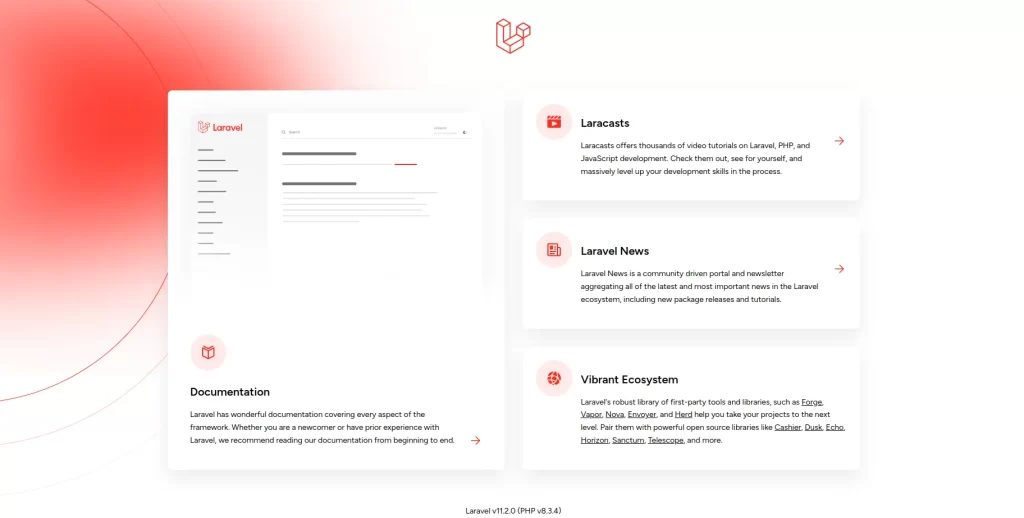
Connect With Database
Create database, user, password and replace below information with yours.
DB_DATABASE=blog
DB_USERNAME=root
DB_PASSWORD=password
Migrate Laravel App
If you using Highier version of laravel, you have to run migration to create needable database.
php artisan migrate
What are Models in Laravel Framework and How to Use it
A Model is an PHP class in laravel framework that represent the database table. Basically It is responsible for maintaining the data in table and giving a method to user to interact with it. Data can be updated, added, retrieved or fetching and finally deleted by this models. So, let’s consider model is an manager of your data, they gurantee the validity, accuracy and safety of your data, and You can develop an User Model in Laravel if your database contains User Table. Generally the User model would be the manager to getting user records out of the database, adding new records, updating already existing records and deleting existing records.
Identically models are written as extension to the Eloquent ORM(Object-Relational Mapping) architecture in the form of PHP classes. By enabling developers to interacts with database tables. Further if you want to make model you can manually create a PHP file in the app/Models directory or use the Command make:model
Artisan command to generates a Laravel Model automatically. In order to perform CRUD(Create, Read, Update and Delete) operations on database records, But you must have to create Model. Use all() the method on the Model, for instance to get every records from table.
Create Model
Surely now we are going to write an model Post
to connect with database.
<?php
namespace App\Models;
use App\Models\User;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $table = ('posts');
protected $guarded = [];
protected static function boot()
{
parent::boot();
static::deleting(function ($post) {
});
}
public function user()
{
return $this->belongsTo(User::class);
}
public function scopePublished($query)
{
return $query->where('is_published', true);
}
public function scopeDrafted($query)
{
return $query->where('is_published', false);
}
public function getPublishedAttribute()
{
return ($this->is_published) ? 'Yes' : 'No';
}
public function getEtagAttribute()
{
return hash('sha256', "product-{$this->id}-{$this->updated_at}");
}
}
What are Controllers in Laravel Framework and How to Use it
Controllers are an necessary features in Laravel framework. Undoubtedly a controller is c in model-view-controller(MVC), Laravel is an MVC framework. Firstly controllers are classes that contains functions and methods to handle various HTTP requests. Laravel controllers are used to handle request within the single class, and the controllers are defined in app/http/controllers
directory. Secondly most of the application logics are written in Laravel Controller. i.e. we can create as many controller as needed for our applications for making our application code to have an easy-to-understand syntax. Creating controllers automatically by using this command php artisan make:controller ControllerName
.
Create Controller for Professional Blog
So let’s create a controller named BlogController.php
to display articles list and current articles on page.
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Post;
class BlogController extends Controller
{
}
Create Index Function
Create index function to show list of all the articles.
public function index()
{
$posts = Post::get()->all();
$title = 'Blog | Luis N Vaya | Top Modular Kitchen Service Providers';
return view('posts.index', compact('posts', 'title'));
}
Now Let’s create an function to show articles.
public function post($id)
{
$postquery = Post::orderBy('id','DESC');
$postquery->where('slug',$id);
$post = $postquery->first();
$title = $post->title;
return view('posts.show-blog', compact('post', 'title'));
}
Here is the full code snippet of BlogController
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\Models\Post;
class BlogController extends Controller
{
public function index()
{
$posts = Post::get()->all();
$title = 'Blog | Luis N Vaya | Top Modular Kitchen Service Providers';
return view('posts.index', compact('posts', 'title'));
}
public function post($id)
{
$postquery = Post::orderBy('id','DESC');
$postquery->where('slug',$id);
$post = $postquery->first();
$title = $post->title;
return view('posts.show-blog', compact('post', 'title'));
}
}
Create PostController Controller
Create PostController.php
to create, edit, publish and delete the articles.
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Support\Str;
use Illuminate\Http\Request;
use App\Http\Requests\PostRequest;
use Illuminate\Support\Facades\Cache;
use App\Http\Controllers\Controller;
class PostController extends Controller
{
// write your code here
}
Create Index Function to List All The Article
So, let’s create a public function to serve all the articles list in a table.
public function index()
{
$posts = Post::with(['user'])->paginate(10);
return view('dashboard.posts.index', compact('posts'));
}
Create Create
Function to Create New Article
Create function redirect to create article page. It’s just an function
public function create()
{
return view('dashboard.posts.create');
}
Create Store Function to Save new Article
public function store(Request $request)
{
// $this->validate($request, [
// 'name'=>'required|min:2',
// 'fname'=>'required|min:2',
// 'enrollment'=>'required',
// 'mobile'=>'required|min:10',
// 'email'=>'required|unique:tbl_student',
// 'yearOfEnrollment'=>'required',
// 'postName'=>'required',
// ]);
$data = $request->all();
$path = 'assets/post/';
$destinationPath = $path;
$image_file =$request->file('images');
$image = '';
if($image_file){
$file_size = $image_file->getSize();
$image_name = $image_file->getClientOriginalName();
$extention = $image_file->getClientOriginalExtension();
$image = value(function() use ($image_file){
$filename = time().'.'. $image_file->getClientOriginalExtension();
return strtolower($filename);
});
$image_file->move($destinationPath, $image);
}
$post = Post::create([
'title' => $data['title'],
'body' => $data['body'],
'meta_tag' => $data['meta_tag'],
'meta_description' => $data['meta_description'],
'slug' => Str::slug($data['title']),
'keywords' => $data['keywords'],
'image' => $image,
'tags' => $data['tags']
]);
if(isset($post)) {
return redirect()->route('posts.index')->with('message','Student successfully added.');
}else{
return redirect()->back();
}
}
Create Show Function to Display The Article
public function show(Post $post)
{
$post = $post->load(['user']);
return view('dashboard.posts.show', compact('post'));
}
Create Edit Function to Edit The Article
public function edit(Post $post)
{
return view('dashboard.posts.edit', compact('post'));
}
Create Update Function to Update The Article
public function update(Request $request, $id)
{
// $this->validate($request, [
// 'email'=>'required|unique:tbl_student,email,'.$id,
// 'name'=>'required|min:2',
// 'fname'=>'required|min:2',
// 'enrollment'=>'required',
// 'mobile'=>'required|min:10',
// 'yearOfEnrollment'=>'required',
// 'postName'=>'required',
// ]);
$post = Post::findOrFail($id);
$path = 'assets/post/';
$destinationPath = $path;
$image_file =$request->file('images');
$image = '';
if($image_file){
$file_size = $image_file->getSize();
$image_name = $image_file->getClientOriginalName();
$extention = $image_file->getClientOriginalExtension();
$image = value(function() use ($image_file){
$filename = time().'.'. $image_file->getClientOriginalExtension();
return strtolower($filename);
});
$image_file->move($destinationPath, $image);
$post->image = $image;
}
$post->title = $request->input('title');
$post->body = $request->input('body');
$post->meta_tag = $request->input('meta_tag');
$post->meta_description = $request->input('meta_description');
$post->slug = Str::slug($request->input('title'));
$post->keywords = $request->input('keywords');
$post->tags =$request->input('tags');
$upate = $post->save();
if(isset($upate)) {
return redirect()->route('posts.index')->with('message','Posts successfully Updated.');
}else{
return redirect()->back();
}
}
Create Destroy Function to Delete The Article
public function destroy($id)
{
$post = Post::findOrFail($id);
$delete = $post->delete();
if(isset($delete)) {
return redirect()->route('posts.index');
}else{
return redirect()->back();
}
}
Create Publish Function to publish The Article
public function publish(Post $post)
{
$post->is_published = !$post->is_published;
$post->save();
return redirect()->route('posts.index');
}
PostController.php
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Support\Str;
use Illuminate\Http\Request;
use App\Http\Requests\PostRequest;
use Illuminate\Support\Facades\Cache;
use App\Http\Controllers\Controller;
class PostController extends Controller
{
public function index()
{
$posts = Post::with(['user'])->paginate(10);
return view('dashboard.posts.index', compact('posts'));
}
public function create()
{
return view('dashboard.posts.create');
}
public function store(Request $request)
{
// $this->validate($request, [
// 'name'=>'required|min:2',
// 'fname'=>'required|min:2',
// 'enrollment'=>'required',
// 'mobile'=>'required|min:10',
// 'email'=>'required|unique:tbl_student',
// 'yearOfEnrollment'=>'required',
// 'postName'=>'required',
// ]);
$data = $request->all();
$path = 'assets/post/';
$destinationPath = $path;
$image_file =$request->file('images');
$image = '';
if($image_file){
$file_size = $image_file->getSize();
$image_name = $image_file->getClientOriginalName();
$extention = $image_file->getClientOriginalExtension();
$image = value(function() use ($image_file){
$filename = time().'.'. $image_file->getClientOriginalExtension();
return strtolower($filename);
});
$image_file->move($destinationPath, $image);
}
$post = Post::create([
'title' => $data['title'],
'body' => $data['body'],
'meta_tag' => $data['meta_tag'],
'meta_description' => $data['meta_description'],
'slug' => Str::slug($data['title']),
'keywords' => $data['keywords'],
'image' => $image,
'tags' => $data['tags']
]);
if(isset($post)) {
return redirect()->route('posts.index')->with('message','Student successfully added.');
}else{
return redirect()->back();
}
}
public function show(Post $post)
{
$post = $post->load(['user']);
return view('dashboard.posts.show', compact('post'));
}
public function edit(Post $post)
{
return view('dashboard.posts.edit', compact('post'));
}
public function update(Request $request, $id)
{
// $this->validate($request, [
// 'email'=>'required|unique:tbl_student,email,'.$id,
// 'name'=>'required|min:2',
// 'fname'=>'required|min:2',
// 'enrollment'=>'required',
// 'mobile'=>'required|min:10',
// 'yearOfEnrollment'=>'required',
// 'postName'=>'required',
// ]);
$post = Post::findOrFail($id);
$path = 'assets/post/';
$destinationPath = $path;
$image_file =$request->file('images');
$image = '';
if($image_file){
$file_size = $image_file->getSize();
$image_name = $image_file->getClientOriginalName();
$extention = $image_file->getClientOriginalExtension();
$image = value(function() use ($image_file){
$filename = time().'.'. $image_file->getClientOriginalExtension();
return strtolower($filename);
});
$image_file->move($destinationPath, $image);
$post->image = $image;
}
$post->title = $request->input('title');
$post->body = $request->input('body');
$post->meta_tag = $request->input('meta_tag');
$post->meta_description = $request->input('meta_description');
$post->slug = Str::slug($request->input('title'));
$post->keywords = $request->input('keywords');
$post->tags =$request->input('tags');
$upate = $post->save();
if(isset($upate)) {
return redirect()->route('posts.index')->with('message','Posts successfully Updated.');
}else{
return redirect()->back();
}
}
public function destroy($id)
{
$post = Post::findOrFail($id);
$delete = $post->delete();
if(isset($delete)) {
return redirect()->route('posts.index');
}else{
return redirect()->back();
}
}
public function publish(Post $post)
{
$post->is_published = !$post->is_published;
$post->save();
return redirect()->route('posts.index');
}
}
What is web.php in Laravel framework?
All laravel routes are defined in web.php file, which is located in routes/web.php
. web.php
is an file where we write our applications url’s to be visited by application users. There are overall 4 types of routes methods GET, PUT, POST and DELETE. In reality laravel framework maps all the requests with the help of routes. Unquestionably all the routes are associated with specific controller.
Create Route for Blog
Let’s create routes for user view blog and backend posts. I am going to create two routes one for blog list and one for detailed blog view.
// routes for frontend to display blog
Route::get('/blog', [App\Http\Controllers\BlogController::class, 'index'])->name('blog_list');
Route::get('/blog/{id}', [App\Http\Controllers\BlogController::class, 'post'])->name('blogs');
// routes for backend
Route::resource('/posts', 'App\Http\Controllers\PostController');
Route::get('posts/{id}/delete','App\Http\Controllers\PostController@destroy');
Route::get('/posts/{post}/publish','App\Http\Controllers\PostController@publish');
What is view in MVC in laravel php
Generally view is the V in MVC framework, that contains HTML markups, php code and blade template. Laravel uses blade template engine to serve view. In brief all views templates are stored in Laravel projects resources/views
directory. Basically laravel view is an php file that is responsible for delivering the user interface of your web applications. In fact it provides a way to display data to the user and allow the user to interact with the application.
In other words we can say it’s an front-end of your application. They insure how your application looks and feel to the user, in general it enhance better user experiences. i.e. you have a page that displays a list of articles, you could create a Article View in Laravel. In a word the Articial View would contain HTML and PHP code that display the list of articles retrieved from Articles Model. Meanwhile laravel view have .blade.php
extension.
Create View
Then let’s create posts
directory in resources
directory and create index.blade.php
, show-blog.blade.php
and blog_master.blade.php
blog_master.blade.php
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>{{ $title }}</title>
<meta name="robots" content="index, follow" >
<meta name="description" content="Luisnvaya - ">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1">
<meta name="msvalidate.01" content="2831CC3FF80C61E18759E18201B9E6E0" />
<meta name="google-site-verification" content="w8DEWLWZyrAf9x3t3FqDVltDdXacFqYZTUJNNQIREYs" />
@yield('additional_meta')
<link rel="shortcut icon" type="image/x-icon" href="images/favicon.png">
<!-- STYLES -->
<link rel="stylesheet" href="{{asset('assets/blog/css/bootstrap.min.css')}}" type="text/css" media="all">
<link rel="stylesheet" href="{{asset('assets/blog/css/all.min.css')}}" type="text/css" media="all">
<link rel="stylesheet" href="{{asset('assets/blog/css/slick.css')}}" type="text/css" media="all">
<link rel="stylesheet" href="{{asset('assets/blog/css/simple-line-icons.css')}}" type="text/css" media="all">
<link rel="stylesheet" href="{{asset('assets/blog/css/style.css')}}" type="text/css" media="all">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css">
@yield('additional_static')
@yield('extracss')
</head>
<body>
<!-- preloader -->
<div id="preloader">
<div class="book">
<div class="inner">
<div class="left"></div>
<div class="middle"></div>
<div class="right"></div>
</div>
<ul>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
</div>
</div>
<!-- site wrapper -->
<div class="site-wrapper">
<div class="main-overlay"></div>
<!-- header -->
@include('posts.utils.blog_header')
@yield('content')
<!-- footer -->
@include('posts.utils.footer')
</div><!-- end site wrapper -->
<!-- search popup area -->
@include('posts.utils.search')
<!-- side menu -->
@include('posts.utils.canva')
<!-- JAVA SCRIPTS -->
<script src="{{asset('assets/blog/js/jquery.min.js')}}"></script>
<script src="{{asset('assets/blog/js/popper.min.js')}}"></script>
<script src="{{asset('assets/blog/js/bootstrap.min.js')}}"></script>
<script src="{{asset('assets/blog/js/slick.min.js')}}"></script>
<script src="{{asset('assets/blog/js/jquery.sticky-sidebar.min.js')}}"></script>
<script src="{{asset('assets/blog/js/custom.js')}}"></script>
<!-- <script src='https://cdn.ckeditor.com/4.17.1/standard/ckeditor.js'></script> -->
<script src="https://cdn.tiny.cloud/1/invalid-origin/tinymce/5.4.2-90/tinymce.min.js" referrerpolicy="origin"></script>
<script type="text/javascript">
const url='<?php echo URL('/'); ?>';
</script>
<script>
tinymce.init({
selector: 'textarea',
plugins: 'advlist autolink lists link image charmap print preview hr anchor pagebreak',
toolbar_mode: 'floating',
});
</script>
</body>
</html>
index.blade.php
@extends('posts.blog_master')
@section('description', '')
@section('keywords', '')
@section('content')
<section class="main-content">
<p class="codewithronny" hidden>This website is developed by <a href="https://codewithronny.com/">CodeWithRonny</a></p>
<div class="container-xll padding-30 rounded">
<div class="row" style="align: middle;">
<div class="col-lg-11">
<!-- section header -->
<div class="section-header">
<center><h3 class="section-title">Latest Posts</h3> <img src="{{asset('assets/blog/images/wave.svg')}}" class="wave" alt="wave" /></center>
</div>
<div class="padding-30 rounded bordered">
<div class="row">
@foreach($posts as $post)
<div class="col-md-12 col-sm-6">
<!-- post -->
<div class="post post-list clearfix">
<div class="thumb rounded">
<span class="post-format-sm">
<i class="icon-picture"></i>
</span>
<a href="{{route('blogs',$post->slug)}}">
<div class="inner">
@if($post->image)
<img src="{{asset ('assets/post/' .$post->image )}}" class="img-fluid rounded mt-3" alt="{{ $post->title }}" />
@else
<img src="{{asset('assets/blog/images/posts/latest-sm-1.jpg')}}" alt="{{ $post->title }}" />
@endif
</div>
</a>
</div>
<div class="details">
<ul class="meta list-inline mb-3">
<li class="list-inline-item"><a href="#"><i class="fa-solid fa-user"></i>{{$post->author}}</a></li>
<li class="list-inline-item">{{$post->created_at}}</li>
</ul>
<h5 class="post-title"><a href="{{route('blogs',$post->slug)}}">{{$post->title}}</a></h5>
<!-- <p class="excerpt mb-0">{!! $post->body !!}</p> -->
<div class="post-bottom clearfix d-flex align-items-center">
<div class="social-share me-auto">
<button class="toggle-button icon-share"></button>
<ul class="icons list-unstyled list-inline mb-0">
<li class="list-inline-item"><a href="#"><i class="fab fa-facebook-f"></i></a></li>
<li class="list-inline-item"><a href="#"><i class="fab fa-twitter"></i></a></li>
<li class="list-inline-item"><a href="#"><i class="fab fa-linkedin-in"></i></a></li>
<li class="list-inline-item"><a href="#"><i class="fab fa-pinterest"></i></a></li>
<li class="list-inline-item"><a href="#"><i class="fab fa-telegram-plane"></i></a></li>
<li class="list-inline-item"><a href="#"><i class="far fa-envelope"></i></a></li>
</ul>
</div>
<div class="more-button float-end">
<a href="{{route('blogs',$post->slug)}}"><span class="icon-options"></span></a>
</div>
</div>
</div>
</div>
</div>
@endforeach
</div>
<!-- load more button -->
<div class="text-center">
<button class="btn btn-simple">Load More</button>
</div>
</div>
</div>
</div>
</div>
</section>
@endsection
@section('additional_static')
@endsection
show-blog.blade.php
@extends('posts.blog_master2')
@section('description', '')
@section('keywords', '')
@section('block')
<meta name="name" content="{{$post->meta_title}}" >
<meta name="description" content="{{$post->meta_description}}" >
<meta name="tag" content="{{ $post->tags }}">
<meta name="author" content="Luisnvaya">
<meta name="keywords" content="{{$post->tags}}" >
<meta property="og:title" content="{{$post->title}}">
<meta name="twitter:title" content="{{$post->title}}">
@endsection
@if($post->img)
<meta property="og:image" content="{{$post->img.url}}">
<meta name="twitter:image" content={{$post->img.url}}>
@endif
@section('content')
<section>
@include('posts.utils.blog_header2')
<div class="container-fluid">
<div class="row">
<div class="col-12 col-lg-6 col-xl-8 offset-lg-3 offset-xl-2 py-7 py-lg-9 px-lg-7">
<br><br>
<!-- Heading -->
<h1 class="mb-1 display-4 text-center">{{ $post->title }}</h1><br><br>
<div class="col ml-n5">
<div class="row align-items-center py-5 border-top border-bottom">
<div class="col-auto">
<!-- Avatar -->
<div class="avatar avatar-lg">
<img src="{{asset ('assets/luisnvaya_favicon.png')}}" alt="Luisnvaya favicon" class="avatar-img rounded-circle">
</div>
</div>
<div class="col ml-n5">
<!-- Name -->
<h6 class="text-uppercase mb-0">
Admin
</h6>
<!-- Date -->
<time class="font-size-sm text-muted" datetime="2019-05-20">
Published on {{ $post->updated_at }}
</time>
</div>
<div class="col-auto">
<!-- Share -->
<span class="h6 text-uppercase text-muted d-none d-md-inline mr-4">
Share:
</span>
<!-- Icons -->
<ul class="d-inline list-unstyled list-inline list-social">
<li class="list-inline-item list-social-item mr-3">
<a href="https://www.instagram.com" class="text-decoration-none" target="_blank">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/instagram.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/facebook.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/twitter.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
</ul>
</div>
</div>
<br><br>
<div class="row justify-content-center">
<div class="col-12 col-md-10 col-lg-11 col-xl-11">
@if($post->image)
<figure class="figure mb-7">
<!-- Image -->
<img class="figure-img img-fluid rounded lift lift-lg" src="{{asset ('assets/post/' .$post->image )}}" alt="{{ $post->title }}">
<!-- Caption -->
<!-- <figcaption class="figure-caption text-center">
This is a caption on this photo for reference
</figcaption> -->
</figure>
@endif
<p class="lead mb-7 text-center text-muted">
{!! $post->body !!}
</p>
</div>
<p><strong>Tags: </strong>{{ $post->tags }}</p>
</div>
<br><br>
<div class="row align-items-center py-5 border-top border-bottom">
<div class="col-auto">
<!-- Avatar -->
<div class="avatar avatar-lg">
<img src="{{asset ('assets/luisnvaya_favicon.png')}}" alt="..." class="avatar-img rounded-circle">
</div>
</div>
<div class="col ml-n5">
<!-- Name -->
<h6 class="text-uppercase mb-0">
Admin
</h6>
<!-- Date -->
<time class="font-size-sm text-muted" datetime="2019-05-20">
Published on {{ $post->updated_at }}
</time>
</div>
<div class="col-auto">
<!-- Share -->
<span class="h6 text-uppercase text-muted d-none d-md-inline mr-4">
Share:
</span>
<!-- Icons -->
<ul class="d-inline list-unstyled list-inline list-social">
<li class="list-inline-item list-social-item mr-3">
<a href="https://www.instagram.com" class="text-decoration-none" target="_blank">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/instagram.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/facebook.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/twitter.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
</ul>
</div>
</div>
</div>
</div> <!-- / .row -->
</div>
</section>
@endsection
@section('additional_static')
@endsection
After all inside resources
directory create dashboard/posts
directory.
create _form.blade.php
<textarea name="body" id="body" rows="25" style="height:500, ">{{old('body')?old('body'):$body}}</textarea>
<div class="text text-danger" id="bodyErro"></div>
create index.blade.php
@extends('dashboard.layouts.master2')
@section('title', 'Pst List | Luis N Vaya ')
@section('content')
<section class="pt-8 pt-md-11">
<div class="container">
<div class="row align-items-center">
<div class="col-12 col-md">
<!-- Link -->
<a href="" class="font-weight-bold font-size-sm text-decoration-none mb-3">
<i class="fe fe-arrow-left mr-3"></i> Back
</a>
</div>
<div class="col-auto">
<!-- Buttons -->
<a href="{{route('posts.create')}}" class="btn btn-primary">
Write post
</a>
</div>
</div> <!-- / .row -->
</div><!-- / .container -->
</section>
<div class="row">
<div class="col-12">
<!-- Divider -->
<hr class=" border-gray-300">
</div>
</div> <!-- / .row -->
<section class="pt-6 pt-md-8">
<div class="container pb-8 pb-md-11 border-bottom border-gray-300">
<div class="row">
<div class="col-12">
<div class="row">
<div class="col-12 col-md-12">
<!-- Table -->
<div class="table-responsive mb-7 mb-md-9">
<table class="table table-align-right">
<thead>
<tr>
<th>
<span class="h6 text-uppercase font-weight-bold">
Title
</span>
</th>
<th>
<span class="h6 text-uppercase font-weight-bold">
Body
</span>
</th>
<th>
<span class="h6 text-uppercase font-weight-bold">
Tags
</span>
</th>
<th>
<span class="h6 text-uppercase font-weight-bold">
Published
</span>
</th>
<th>
<span class="h6 text-uppercase font-weight-bold">
Action
</span>
</th>
</tr>
</thead>
<tbody>
@forelse ($posts as $post)
<tr>
<td><span style="font-size:18px;">{{ $post->title }}</span></td>
<td><span style="font-size:18px;">{!! Illuminate\Support\Str::limit($post->body, 100) !!}</span></td>
<td><span style="font-size:18px;">{{ $post->tags }}</span></td>
<td><span style="font-size:18px;">{{ $post->published }}</span></td>
<td>
@can ('blog-list')
@php
if($post->published == 'Yes') {
$label = 'Draft';
} else {
$label = 'Publish';
}
@endphp
<a href="{{ url("/posts/{$post->id}/publish") }}" data-method="PUT" data-token="{{ csrf_token() }}" data-confirm="Are you sure?" class="btn btn-xs btn-warning">{{ $label }}</a>
@endcan
<a href="{{ url("/posts/{$post->id}") }}" class="btn btn-xs btn-success"><span class="fa fa-eye author-log-ic"></span> Show</a>
<a href="{{ url("/posts/{$post->id}/edit") }}" class="btn btn-xs btn-info"><span class="fa fa-edit author-log-ic"></span> Edit</a>
<a href="{{ url("/posts/{$post->id}/delete") }}" data-method="DELETE" data-token="{{ csrf_token() }}" data-confirm="Are you sure?" class="btn btn-xs btn-danger"><span class="fa fa-trash author-log-ic"></span> Delete</a>
</td>
</tr>
@empty
<tr>
<td colspan="5">No post available.</td>
</tr>
@endforelse
</tbody>
</table>
</div>
</div>
</div> <!-- / .row -->
</div>
</div>
</div>
</div>
@endsection
create create.blade.php
@extends('dashboard.layouts.master2')
@section('title', 'Post Create | Luis N Vaya ')
@section('content')
<section class="pt-8 pt-md-11">
<form class="form-horizontal" action="{{ url('posts') }}" method="POST" enctype="multipart/form-data" autocomplete="off">
@csrf
<?php
$title ="";
$body ="";
$meta_tag ="";
$meta_description ="";
$slug ="";
$keywords ="";
$tags = '';
?>
<div class="container">
<div class="row align-items-center">
<div class="col-12 col-md">
<!-- Link -->
<a href="{{ route('posts.index') }}" class="font-weight-bold font-size-sm text-decoration-none mb-3">
<i class="fe fe-arrow-left mr-3"></i> Back
</a>
<!-- Heading -->
<!-- <h1 class="display-4 mb-2">
</h1> -->
<!-- Text -->
<!-- <p class="font-size-lg text-gray-700 mb-5 mb-md-0">
</p> -->
</div>
<div class="col-auto">
<!-- Buttons -->
<a href="{{ route('posts.index') }}" class="btn btn-primary-soft mr-1">
Cancel
</a>
<button type="submit" class="btn btn-primary">
Update
</button>
</div>
</div> <!-- / .row -->
</div><!-- / .container -->
<div class="row">
<div class="col-12">
<!-- Divider -->
<hr class=" border-gray-300">
</div>
</div> <!-- / .row -->
<div class="row">
<div class="col-12 col-md-9">
@include('dashboard.posts._form')
</div>
<div class="col-12 col-md-3">
<div class="">
<div class="collapse d-lg-block" id="sidenavCollapse">
<div class="">
<!-- Heading -->
<h6 class="text-uppercase font-weight-bold">
Title
</h6>
<!-- Links -->
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="title" value="{{old('title')?old('title'):$title}}" placeholder="" />
<div class="text text-danger" id="titleErro"></div>
</li>
</ul>
<!-- Heading -->
<h6 class="text-uppercase font-weight-bold">
permalink
</h6>
<!-- Links -->
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="slug" value="{{old('slug')?old('slug'):$slug}}" placeholder="Enter Slug" />
<div class="text text-danger" id="slugErro"></div>
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Image
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="file" name="images" />
<div class="text text-danger" id="imagesErro"></div>
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Tag
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="tags" value="{{old('tags')?old('tags'):$tags}}" placeholder="" />
<div class="text text-danger" id="tagsErro"></div>
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Meta Tag
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="meta_tag" value="{{old('meta_tag')?old('meta_tag'):$meta_tag}}" placeholder="" />
<div class="text text-danger" id="meta_tagErro"></div>
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Meta Description
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="meta_description" value="{{old('meta_description')?old('meta_description'):$meta_description}}" placeholder="" />
<div class="text text-danger" id="meta_descriptionErro"></div>
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Focus Keywords
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="keywords" value="{{old('keywords')?old('keywords'):$keywords}}" placeholder="" />
<div class="text text-danger" id="keywordsErro"></div>
</li>
</ul>
</div>
</div>
</div>
</div>
</div> <!-- / .row -->
</form>
</section>
<script type="text/javascript">
function readURL(input) {
if (input.images && input.images[0]) {
var reader = new FileReader();
reader.onload = function(e) {
$('#imagePreview').attr('src', e.target.result);
}
reader.readAsDataURL(input.files[0]);
}
}
</script>
@endsection
create edit.blade.php
@extends('dashboard.layouts.master2')
@section('title', 'Post Update | Luis N Vaya ')
@section('content')
<section class="pt-8 pt-md-11">
<form class="form-horizontal" action="{{ url('posts/' . $post->id) }}" method="POST" enctype="multipart/form-data" autocomplete="off">
@csrf
@method('PUT')
<?php
$title = $post->title;
$body = $post->body;
$meta_tag = $post->meta_tag;
$meta_description = $post->meta_description;
$slug = $post->slug;
$keywords = $post->keywords;
$tags = $post->tags;
?>
<div class="container">
<div class="row align-items-center">
<div class="col-12 col-md">
<!-- Link -->
<a href="{{ route('posts.index') }}" class="font-weight-bold font-size-sm text-decoration-none mb-3">
<i class="fe fe-arrow-left mr-3"></i> Back
</a>
<!-- Heading -->
<!-- <h1 class="display-4 mb-2">
</h1> -->
<!-- Text -->
<!-- <p class="font-size-lg text-gray-700 mb-5 mb-md-0">
</p> -->
</div>
<div class="col-auto">
<!-- Buttons -->
<a href="{{ route('posts.index') }}" class="btn btn-primary-soft mr-1">
Cancel
</a>
<button type="submit" class="btn btn-primary">
Update
</button>
</div>
</div> <!-- / .row -->
</div><!-- / .container -->
<div class="row">
<div class="col-12">
<!-- Divider -->
<hr class=" border-gray-300">
</div>
</div> <!-- / .row -->
<div class="row">
<div class="col-12 col-md-9">
@include('dashboard.posts._form')
</div>
<div class="col-12 col-md-3">
<div class="">
<div class="collapse d-lg-block" id="sidenavCollapse">
<div class="">
<!-- Heading -->
<h6 class="text-uppercase font-weight-bold">
Title
</h6>
<!-- Links -->
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="title" value="{{old('title')?old('title'):$title}}" placeholder="" />
<div class="text text-danger" id="titleErro"></div>
@if($errors->has('title'))
<div class="text text-danger">{{ $errors->first('title') }}</div>
@endif
</li>
</ul>
<!-- Heading -->
<h6 class="text-uppercase font-weight-bold">
permalink
</h6>
<!-- Links -->
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="slug" value="{{old('slug')?old('slug'):$slug}}" placeholder="Enter Slug" />
<div class="text text-danger" id="slugErro"></div>
@if($errors->has('slug'))
<div class="text text-danger">{{ $errors->first('slug') }}</div>
@endif
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Image
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="file" name="images" />
<div class="text text-danger" id="imagesErro"></div>
@if($errors->has('images'))
<div class="text text-danger">{{ $errors->first('images') }}</div>
@endif
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Tag
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="tags" value="{{old('tags')?old('tags'):$tags}}" placeholder="" />
<div class="text text-danger" id="tagsErro"></div>
@if($errors->has('tags'))
<div class="text text-danger">{{ $errors->first('tags') }}</div>
@endif
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Meta Tag
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="meta_tag" value="{{old('meta_tag')?old('meta_tag'):$meta_tag}}" placeholder="" />
<div class="text text-danger" id="meta_tagErro"></div>
@if($errors->has('meta_tag'))
<div class="text text-danger">{{ $errors->first('meta_tag') }}</div>
@endif
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Meta Description
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="meta_description" value="{{old('meta_description')?old('meta_description'):$meta_description}}" placeholder="" />
<div class="text text-danger" id="meta_descriptionErro"></div>
@if($errors->has('meta_description'))
<div class="text text-danger">{{ $errors->first('meta_description') }}</div>
@endif
</li>
</ul>
<h6 class="text-uppercase font-weight-bold">
Focus Keywords
</h6>
<ul class="list mb-6">
<li class="list-item">
<input type="text" class="form-control" name="keywords" value="{{old('keywords')?old('keywords'):$keywords}}" placeholder="" />
<div class="text text-danger" id="keywordsErro"></div>
@if($errors->has('keywords'))
<div class="text text-danger">{{ $errors->first('keywords') }}</div>
@endif
</li>
</ul>
</div>
</div>
</div>
</div>
</div> <!-- / .row -->
</form>
</section>
<script type="text/javascript">
function readURL(input) {
if (input.images && input.images[0]) {
var reader = new FileReader();
reader.onload = function(e) {
$('#imagePreview').attr('src', e.target.result);
}
reader.readAsDataURL(input.files[0]);
}
}
</script>
@endsection
create show.blade.php
@extends('dashboard.layouts.master2')
@section('title', 'Post Show | Luis N Vaya ')
@section('content')
<a href="{{ route('posts.index') }}" class="btn btn-default pull-right"><- Go Back</a>
<br><br><br>
<!-- SHAPE
================================================== -->
<div class="position-relative">
<div class="shape shape-bottom shape-fluid-x svg-shim text-light">
<svg viewBox="0 0 2880 48" fill="none" xmlns="http://www.w3.org/2000/svg"><path d="M0 48h2880V0h-720C1442.5 52 720 0 720 0H0v48z" fill="currentColor"/></svg>
</div>
</div>
<section>
<div class="container-fluid">
<div class="row">
<div class="col-12 col-lg-6 col-xl-8 offset-lg-3 offset-xl-2 py-7 py-lg-9 px-lg-7">
<br><br>
<!-- Heading -->
<h1 class="mb-1 display-4 text-center">{{ $post->title }}</h1><br><br>
<div class="col ml-n5">
<div class="row align-items-center py-5 border-top border-bottom">
<div class="col-auto">
<!-- Avatar -->
<div class="avatar avatar-lg">
<img src="{{asset ('assets/luisnvaya_favicon.png')}}" alt="..." class="avatar-img rounded-circle">
</div>
</div>
<div class="col ml-n5">
<!-- Name -->
<h6 class="text-uppercase mb-0">
Admin
</h6>
<!-- Date -->
<time class="font-size-sm text-muted" datetime="2019-05-20">
Published on {{ $post->updated_at }}
</time>
</div>
<div class="col-auto">
<!-- Share -->
<span class="h6 text-uppercase text-muted d-none d-md-inline mr-4">
Share:
</span>
<!-- Icons -->
<ul class="d-inline list-unstyled list-inline list-social">
<li class="list-inline-item list-social-item mr-3">
<a href="https://www.instagram.com" class="text-decoration-none" target="_blank">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/instagram.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/facebook.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/twitter.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
</ul>
</div>
</div>
<br><br>
<div class="row justify-content-center">
<div class="col-12 col-md-10 col-lg-11 col-xl-11">
<figure class="figure mb-7">
<!-- Image -->
<img class="figure-img img-fluid rounded lift lift-lg" src="{{asset ('assets/post/' .$post->image )}}" alt="...">
<!-- Caption -->
<!-- <figcaption class="figure-caption text-center">
This is a caption on this photo for reference
</figcaption> -->
</figure>
<p class="lead mb-7 text-center text-muted">
{!! $post->body !!}
</p>
</div>
<p><strong>Tags: </strong>{{ $post->tags }}</p>
</div>
<br><br>
<div class="row align-items-center py-5 border-top border-bottom">
<div class="col-auto">
<!-- Avatar -->
<div class="avatar avatar-lg">
<img src="{{asset ('assets/luisnvaya_favicon.png')}}" alt="..." class="avatar-img rounded-circle">
</div>
</div>
<div class="col ml-n5">
<!-- Name -->
<h6 class="text-uppercase mb-0">
Admin
</h6>
<!-- Date -->
<time class="font-size-sm text-muted" datetime="2019-05-20">
Published on {{ $post->updated_at }}
</time>
</div>
<div class="col-auto">
<!-- Share -->
<span class="h6 text-uppercase text-muted d-none d-md-inline mr-4">
Share:
</span>
<!-- Icons -->
<ul class="d-inline list-unstyled list-inline list-social">
<li class="list-inline-item list-social-item mr-3">
<a href="https://www.instagram.com" class="text-decoration-none" target="_blank">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/instagram.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/facebook.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
<li class="list-inline-item list-social-item mr-3">
<a href="#!" class="text-decoration-none">
<img src="{{asset ('shawosy/assets/ui/img/icons/social/twitter.svg')}}" class="list-social-icon" alt="...">
</a>
</li>
</ul>
</div>
</div>
</div>
</div> <!-- / .row -->
</div>
</section>
@endsection
Inside dashboard
directory create layouts
directory
, now create master2.blade.php
<!doctype html>
<html lang="en">
<meta http-equiv="content-type" content="text/html;charset=utf-8" />
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<link rel="icon" href="{{asset('favicon.ico')}}" type="image/x-icon" />
<!--====== Favicon Icon ======-->
<link rel="shortcut icon" href="{{asset ('shawosy/assets/logo/favicon.png')}}" type="image/png">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="tag" content="@yield('meta_tag')">
<meta name="description" content="@yield('meta_description')">
<meta name="Keywords" content="@yield('keywords')">
<meta name="author" content="@yield('author')">
<!-- Libs CSS -->
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/fonts/Feather/feather.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/%40fancyapps/fancybox/dist/jquery.fancybox.min.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/aos/dist/aos.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/choices.js/public/shawosy/assets/styles/choices.min.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/flickity-fade/flickity-fade.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/flickity/dist/flickity.min.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/highlightjs/styles/vs2015.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/jarallax/dist/jarallax.css')}}">
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/libs/quill/dist/quill.core.css')}}" />
<!-- Map -->
<link href="{{asset ('shawosy/assets/ui/api.mapbox.com/mapbox-gl-js/v0.53.0/mapbox-gl.css')}}" rel='stylesheet' />
<!-- Theme CSS -->
<link rel="stylesheet" href="{{asset ('shawosy/assets/ui/css/theme.min.css')}}">
<title>@yield('title')</title>
</head>
<body >
@yield('content')
<!-- FOOTER
================================================== -->
@yield('extrajs')
<!-- Place the first <script> tag in your HTML's <head> -->
<script src="https://cdn.tiny.cloud/1/tuig8csskih7cnyvijgmfwu1626pqgoo9cwerfxjpustlnzu/tinymce/7/tinymce.min.js" referrerpolicy="origin"></script>
<!-- Place the following <script> and <textarea> tags your HTML's <body> -->
<script>
tinymce.init({
selector: 'textarea',
plugins: 'anchor autolink charmap codesample emoticons image link lists media searchreplace table visualblocks wordcount checklist mediaembed casechange export formatpainter pageembed linkchecker a11ychecker tinymcespellchecker permanentpen powerpaste advtable advcode editimage advtemplate ai mentions tinycomments tableofcontents footnotes mergetags autocorrect typography inlinecss markdown',
toolbar: 'undo redo | blocks fontfamily fontsize | bold italic underline strikethrough | link image media table mergetags | addcomment showcomments | spellcheckdialog a11ycheck typography | align lineheight | checklist numlist bullist indent outdent | emoticons charmap | removeformat',
tinycomments_mode: 'embedded',
tinycomments_author: 'Author name',
mergetags_list: [
{ value: 'First.Name', title: 'First Name' },
{ value: 'Email', title: 'Email' },
],
ai_request: (request, respondWith) => respondWith.string(() => Promise.reject("See docs to implement AI Assistant")),
});
</script>
<!-- JAVASCRIPT
================================================== -->
<!-- Libs JS -->
<script src="{{asset ('shawosy/assets/ui/libs/jquery/dist/jquery.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/bootstrap/dist/js/bootstrap.bundle.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/%40fancyapps/fancybox/dist/jquery.fancybox.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/aos/dist/aos.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/choices.js/public/shawosy/assets/scripts/choices.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/countup.js/dist/countUp.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/dropzone/dist/min/dropzone.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/flickity/dist/flickity.pkgd.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/flickity-fade/flickity-fade.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/highlightjs/highlight.pack.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/imagesloaded/imagesloaded.pkgd.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/isotope-layout/dist/isotope.pkgd.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/jarallax/dist/jarallax.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/jarallax/dist/jarallax-video.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/jarallax/dist/jarallax-element.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/quill/dist/quill.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/smooth-scroll/dist/smooth-scroll.min.js')}}"></script>
<script src="{{asset ('shawosy/assets/ui/libs/typed.js/lib/typed.min.js')}}"></script>
<!-- Map -->
<script src="{{asset ('shawosy/assets/ui/api.mapbox.com/mapbox-gl-js/v0.53.0/mapbox-gl.js')}}"></script>
<!-- Theme JS -->
<script src="{{asset ('shawosy/assets/ui/js/theme.min.js')}}"></script>
</body>
</html>
Replace all the assets with your assets url.
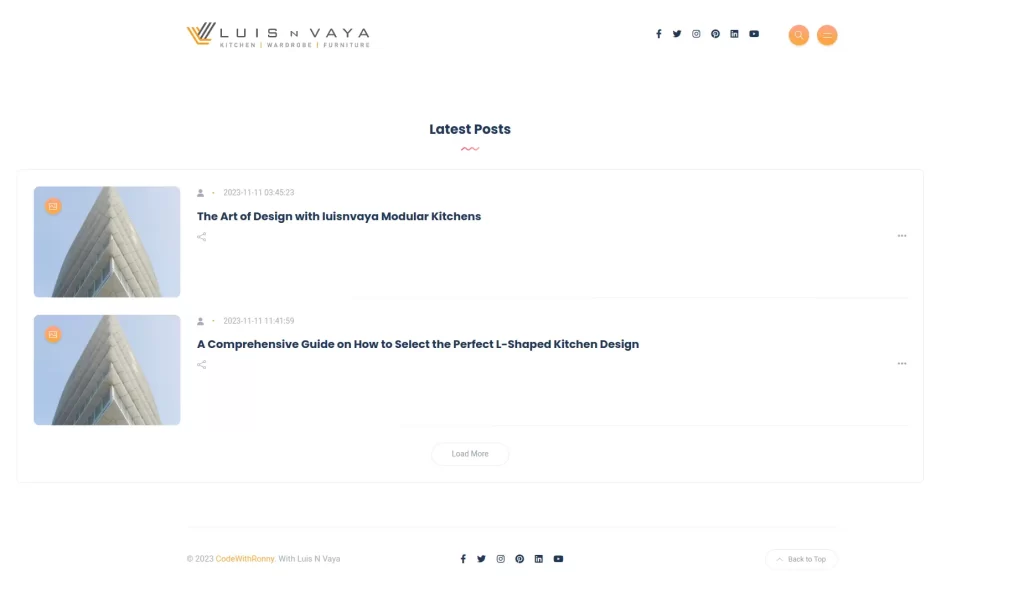
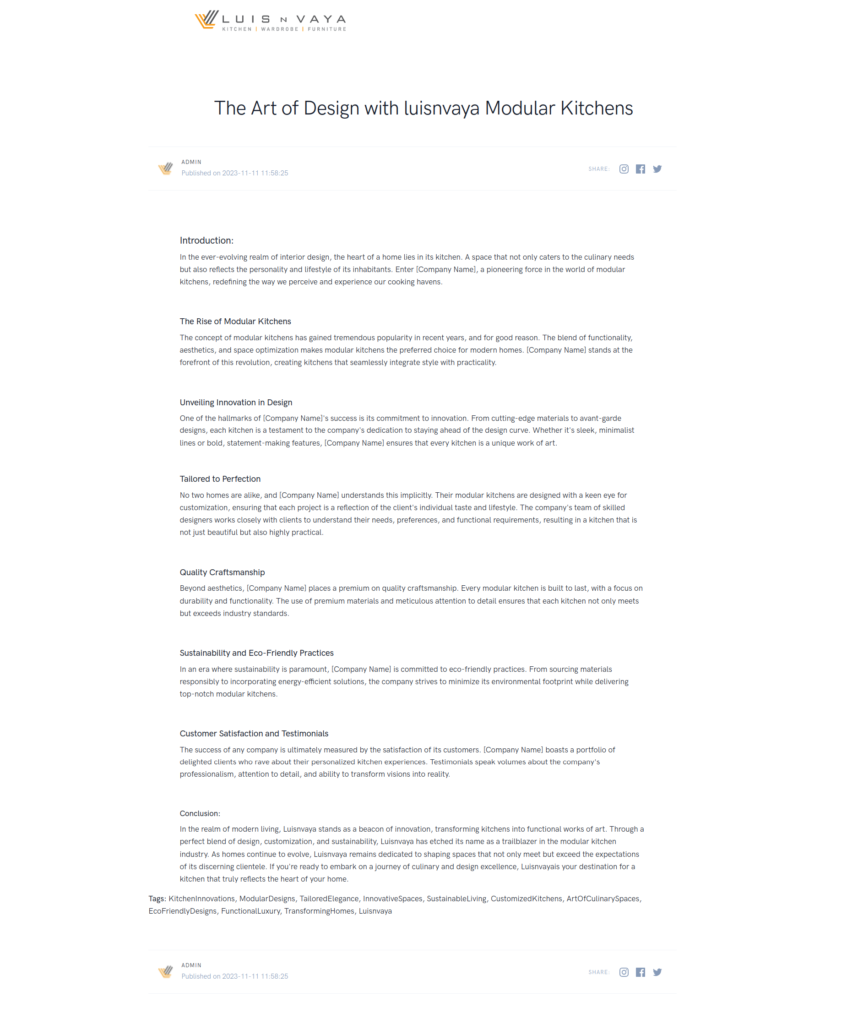
How to create dynamic slider in laravel php
how to run laravel project on localhost
I will guide you step my step to install or run laravel on localhost. But if you already installed it and want to run it locally, use these command php artisan serve
other wise follow these steps:
2. Install Required Dependencies in your system
Make sure you have the following dependency installed:
- PHP (Laravel 11 requires PHP 8.2)
- Composer (PHP dependency manager)
- Database (MySQL, PostgreSQL, SQLite, etc.)
- Node.js & NPM (For frontend assets if needed)
2. Download the Laravel
By use composer to download laravel
composer create-project laravel/laravel name-of-projects
cd name-of-projects
php artisan serve
By using laravel global installer
composer global require laravel/installer
laravel new name-of-projects
cd name-of-projects
php artisan serve
git clone https://github.com/laravel/laravel.git
cd laravel
php artisan serve
Note:–
About Author
I am a Web Developer, Love to write code and explain in brief. I Worked on several projects and completed in no time.
View all posts by Sunil Shaw